Pygame .transform() method
When working with images in pygame, there will be situations where you require real time image manipulation that can be performed in game. Luckily pygame provides built in functionality to enable this, which is the transform()
method.
This method has a few different transformations, but the three most common ones that I regularly use in my games are:
- Scale
- Rotate
- Flip
I will use this dinosaur image for the examples below. It was obtained from itch.io
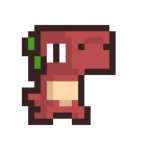
How To Scale Images In Pygame
The scale
method takes in an image and returns a resized version of it. The syntax is below:
resized_image = pygame.transform.scale(image, (new_size))
In this code, the original image is passed in as the first argument. The second argument is the new size in pixels. This is a tuple containing the width and height of the new image.
resized_dino = pygame.transform.scale(dino, (100, 200))
Note that this does not automatically maintain the aspect ratio of the image.
Applying the above code to the dinosaur image resizes it to be 100 pixels wide and 200 pixels tall, resulting in this distorted dino:

In order to maintain the aspect ratio and to scale the image equally, we need to first work out the width and height of the original image, then scale both of those values by the same amount.
w = dino.get_width()
h = dino.get_height()
resized_dino = pygame.transform.scale(dino, (w * 0.5, h * 0.5))
The result is a second image, which is half the size of the original with the same proportions as before.
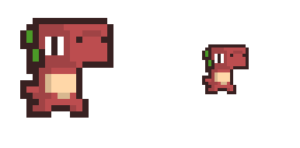
There is an experimental function, scale_by(), which can perform image scaling without working out the width and height. This function was added in pygame 2.1.3 and is shown below.
resized_dino = pygame.transform.scale_by(dino, 0.5)
This function takes the original image as well as the scale factor (in this case I have passed 0.5 so the image will be half the size of the original).
How To Rotate Images In Pygame
The rotate
method takes in only two arguments, the image to be rotated and the angle of rotation (in degrees)
rotated_dino = pygame.transform.rotate(dino, 90)

The result is the image rotated 90 degrees counterclockwise.
How To Flip Images In Pygame
The flip
method allows us to flip an image in the x or y directions (or both). The syntax is shown below. The flip_x
and flip_y
arguments are boolean
values, where True will flip the image in that direction and False won’t.
flipped_image = pygame.transform.flip(image, flip_x, flip_y)
To demonstrate this on the dinosaur we will flip in the x direction.
flipped_dino = pygame.transform.flip(dino, True, False)

The result is the dinosaur image is now facing the left side.
This method is a great way to reduce the number of sprites needed in a game. I use this regularly for character sprites that move left and right. I load in only the images facing in one direction, then I flip the image if I need it to face the other way.
How To Maintain Image Quality With Transform()
One thing to be aware of is that some of these transform
methods – specifically rotate
and scale
– will reduce the quality of the image. This may not be very noticeable initially, but if the same image is scaled or rotated multiple times, it becomes more obvious, resulting in this very sorry looking dinosaur.

To avoid this, instead of modifying the image directly, it is better to create a modified version of it.
This is why in the snippets throughout this guide I have created new variables for the modified image each time I called a transform
method.
May be there is a a bug :
“`python
flipped_image = pygame.transform.rotate(image, flip_x, flip_y)
“`
the method is `flip` , not `rotate`.
By the way , your posts a great and thanks a lot!
Good catch! Thanks for pointing that out.
I have edited the post to correct that section of code.