Why Use Health Bars?
Health bars are found in a lot of games from shooters to platformers, rpgs and many others. They give a quick visual indication of player, enemy or object health allowing the player to react accordingly.
Creating A Health Bar
A health bar can be complex and can include animations, but if we simplify it, the health bar is made up of two rectangles.
- Maximum Health
- Remaining Health
Maximum Health
This rectangle doesn’t change and is always drawn on the screen. This represents the full player health and would typically be red in colour.
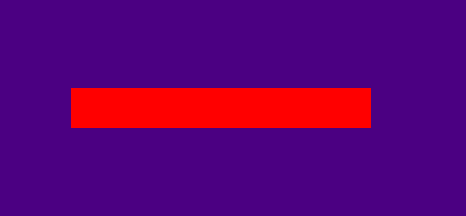
Remaining Health
This rectangle represents the actual amount of health the character or object has. The width of the rectangle is adjusted depending on the remaining health.
At full health, the rectangle is drawn at full width, at half health, the rectangle width is halved, and so on.

Drawing Health Bars
The code for drawing these rectangles uses pygame’s rect
method and both rectangles are drawn on top of each other, with the max health being drawn first.
pygame.draw.rect(screen, "red", (250, 250, 300, 40))
pygame.draw.rect(screen, "green", (250, 250, 300 * ratio, 40))
Notice that the green rectangle width has an additional variable called ratio
.
This is the ratio of remaining health to maximum health and allows us to adjust the width accordingly.
A few examples are shown below to demonstrate.

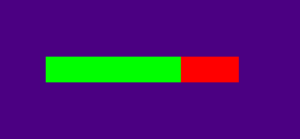
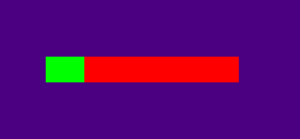
The ratio can be calculated by dividing the remaining health by the maximum health.
max_health = 100
health = 20
ratio = health / max_health
Creating a Health Bar Object
The above code allows you to quickly get started with a health bar, but if you want to create multiple health bars for different objects and characters, it may be easier to use classes.
The health bar can become part of the player’s class or it can be a stand alone class like this.
class HealthBar():
def __init__(self, x, y, w, h, max_hp):
self.x = x
self.y = y
self.w = w
self.h = h
self.hp = max_hp
self.max_hp = max_hp
def draw(self, surface):
#calculate health ratio
ratio = self.hp / self.max_hp
pygame.draw.rect(surface, "red", (self.x, self.y, self.w, self.h))
pygame.draw.rect(surface, "green", (self.x, self.y, self.w * ratio, self.h))
This is similar to the previous code. The __init__
method assigns a few variables to the health bar like its x and y coordinates as well as width and height. It also stores the maximum health.
This means the class can be used to create different health bars with different sizes, positions and healths.
The draw
method includes all the same code from before. It calculates the ratio and draws
the rectangles of the health bar.
To change the amount of health, we simply modify the self.hp variable like so to set it to any value:
health_bar.hp = 50
The final code becomes:
import pygame
pygame.init()
#game window
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 450
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Health Bar')
class HealthBar():
def __init__(self, x, y, w, h, max_hp):
self.x = x
self.y = y
self.w = w
self.h = h
self.hp = max_hp
self.max_hp = max_hp
def draw(self, surface):
#calculate health ratio
ratio = self.hp / self.max_hp
pygame.draw.rect(surface, "red", (self.x, self.y, self.w, self.h))
pygame.draw.rect(surface, "green", (self.x, self.y, self.w * ratio, self.h))
health_bar = HealthBar(250, 200, 300, 40, 100)
run = True
while run:
screen.fill("indigo")
#draw health bar
health_bar.hp = 50
health_bar.draw(screen)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
pygame.display.flip()
pygame.quit()
im glad i found u thanks for ur work to educate us…..so do u have a vid or something on how do i put this health bar on my player
Excellent tutorial you break it down into nice chunks so much easier to understand.
Thanks! Glad to hear it was useful.